Unlocking Database Isolation: A Guide to ACID Properties and Isolation Levels Demystified
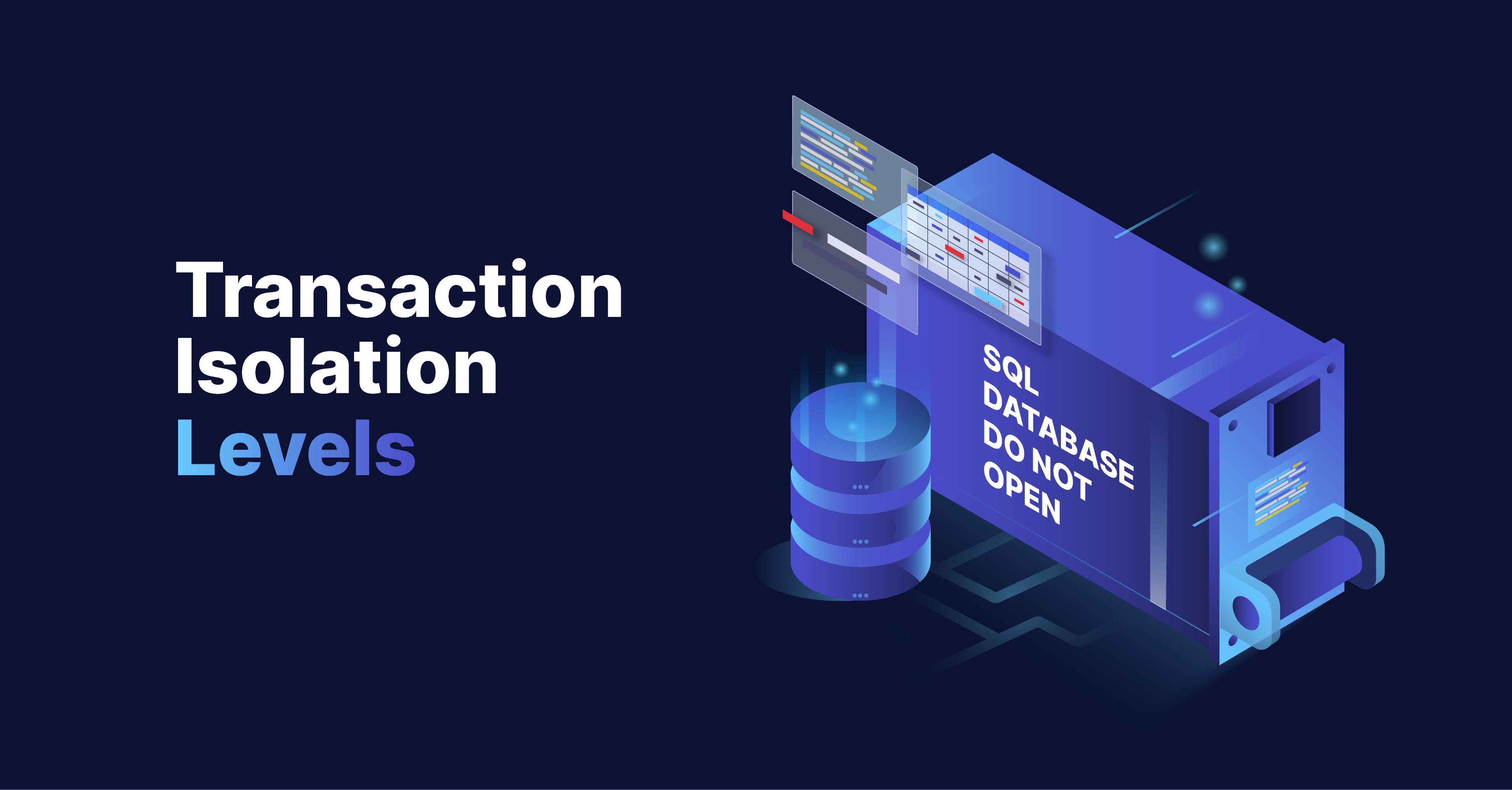
Introduction
In the realm of databases, the ACID properties—Atomicity, Consistency, Isolation, and Durability—constitute the bedrock of reliable and consistent transactions. Isolation, in particular, deals with the concurrent execution of transactions, and various isolation levels define the extent to which transactions are isolated from each other. In this blog post, we will explore these isolation levels with examples and diagrams to better comprehend their impact on database transactions.
1. Read Uncommitted (Level 0)
Description
Read Uncommitted is the lowest isolation level among the ACID properties in database transactions. In this level, transactions are not isolated from each other, meaning that a transaction can read data that has been modified but not yet committed by other transactions. This level allows for a high degree of concurrency, as transactions are not required to wait for others to release locks on the data.
Issues:
- Dirty Reads: Since transactions can read uncommitted changes made by others, there is a risk of encountering dirty reads. A dirty read occurs when one transaction reads data that has been modified by another transaction but not yet committed. If the second transaction is rolled back, the first transaction would have read invalid or "dirty" data.
- Non-repeatable Reads: Another issue is non-repeatable reads, where a transaction reads a piece of data and, when attempting to read the same data again, finds that it has been modified by another transaction in the meantime.
- Phantom Reads: Phantom reads refer to situations where a transaction reads a set of records that satisfy a certain condition, but when attempting to re-read the same set of records, it finds that new records have been inserted by another transaction.
SQL Example:
-- Transaction 1
BEGIN TRANSACTION;
UPDATE products SET stock_quantity = stock_quantity - 10 WHERE product_id = 123;
COMMIT;
-- Transaction 2
BEGIN TRANSACTION;
SELECT * FROM products WHERE product_id = 123; -- May read uncommitted changes
COMMIT;
In this example, if Transaction 2 reads the product data before Transaction 1 commits, it may read the product's stock quantity with the uncommitted changes made by Transaction 1, leading to a dirty read. This lack of isolation can result in data inconsistency and integrity issues.
Diagram:
Transaction 1 Transaction 2
+---------------------+ +---------------------+
1 | Begin Transaction | | Begin Transaction |
+---------------------+ +---------------------+
2 | Update Products | | |
| (Uncommitted) | | |
3 | Commit | | |
+---------------------+ +---------------------+
|
| Select Products
| (Reads Uncommitted)
|
+---------------------+
|
4 | | | Commit |
+---------------------+ +---------------------+
2. Read Committed (Level 1)
Description:
Read Committed is a higher isolation level compared to Read Uncommitted. In this level, transactions are isolated from each other during their execution, meaning a transaction can only read committed data. This provides a higher degree of data consistency compared to Read Uncommitted, as transactions don't read data that is still in the process of being modified.
Issues:
- Non-repeatable Reads: Although Read Committed prevents dirty reads, it still allows for non-repeatable reads. A non-repeatable read occurs when a transaction reads a piece of data, and if another transaction modifies or deletes that data before the first transaction completes, subsequent reads may yield different results.
- Phantom Reads: Similar to Read Uncommitted, Read Committed can still encounter phantom reads. Phantom reads happen when a transaction reads a set of records based on a condition, and another transaction inserts new records that satisfy the same condition, causing the first transaction to perceive a change that wasn't there initially.
SQL Example:
-- Transaction 1
BEGIN TRANSACTION;
UPDATE products SET stock_quantity = stock_quantity - 10 WHERE product_id = 123;
COMMIT;
-- Transaction 2
BEGIN TRANSACTION;
SELECT * FROM products WHERE product_id = 123; -- Reads only committed data
COMMIT;
In this example, Transaction 2 will only read the product data after Transaction 1 commits. However, if Transaction 1 modifies the data between the read and commit phases of Transaction 2, a non-repeatable read may occur. This demonstrates the trade-off between data consistency and concurrency in the Read Committed isolation level.
Diagram:
Transaction 1 Transaction 2
+---------------------+ +---------------------+
1 | Begin Transaction | | Begin Transaction |
+---------------------+ +---------------------+
2 | Update Products | | |
| (Committed) | | |
3 | Commit | | |
+---------------------+ +---------------------+
|
| Select Products
| (Reads Committed)
|
+---------------------+
|
4 | | | Commit |
+---------------------+ +---------------------+
3. Repeatable Read (Level 2)
Description:
Repeatable Read is an isolation level that provides a higher degree of consistency compared to Read Committed. In this level, once a transaction reads a piece of data, it will always see the same data throughout its entire duration, even if other transactions modify or insert new data. This means that if a transaction reads a set of records that satisfy a certain condition, it is guaranteed that those records will not change or be modified by other transactions until the original transaction completes.
Issues:
- Phantom Reads: While Repeatable Read prevents dirty reads and non-repeatable reads, it can still encounter phantom reads. Phantom reads occur when a transaction reads a set of records based on a condition, and another transaction inserts new records that satisfy the same condition. This causes the first transaction to perceive a change that wasn't there initially.
SQL Example:
-- Transaction 1
BEGIN TRANSACTION;
SELECT * FROM products WHERE stock_quantity > 100; -- Prevents changes
COMMIT;
-- Transaction 2
BEGIN TRANSACTION;
UPDATE products SET stock_quantity = stock_quantity + 20 WHERE category = 'Electronics';
COMMIT;
In this example, Transaction 1 reads a set of products with stock quantities greater than 100. The Repeatable Read isolation level ensures that no changes can be made to the data that satisfies this condition until Transaction 1 completes. However, if Transaction 2 inserts new products with stock quantities greater than 100, Transaction 1 might encounter a phantom read in subsequent queries.
Diagram:
Transaction 1 Transaction 2
+---------------------+ +---------------------+
1 | Begin Transaction | | Begin Transaction |
+---------------------+ +---------------------+
2 | Select Products | | |
| (Prevents changes) | | |
3 | Commit | | |
+---------------------+ +---------------------+
|
| Update Products
| (Blocked)
|
+---------------------+
|
4 | | | Commit |
+---------------------+ +---------------------+
4. Serializable (Level 3)
Description:
Serializable is the highest isolation level among the ACID properties of databases. It ensures the strictest form of isolation, making transactions appear as if they are executed in a serial, non-concurrent manner. Serializable transactions are completely isolated from each other, preventing concurrent transactions from reading or modifying the same data simultaneously. This level provides the highest guarantee of data consistency but may come at the cost of reduced concurrency and increased locking.
Issues:
- Reduced Concurrency: Serializable transactions impose strict locking mechanisms to ensure isolation, which can result in reduced concurrency. Transactions may need to wait for locks to be released by other transactions before they can proceed, potentially leading to performance issues in highly concurrent systems.
SQL Example:
-- Transaction 1
BEGIN TRANSACTION;
SELECT * FROM orders WHERE status = 'Pending'; -- Prevents changes by others
COMMIT;
-- Transaction 2
BEGIN TRANSACTION;
UPDATE orders SET status = 'Shipped' WHERE order_id = 456; -- Waits until Transaction 1 completes
COMMIT;
Diagram:
Transaction 1 Transaction 2
+---------------------+ +---------------------+
1 | Begin Transaction | | Begin Transaction |
+---------------------+ +---------------------+
2 | Select Orders | | |
| (Prevents changes) | | |
3 | Commit | | |
+---------------------+ +---------------------+
|
| Update Orders
| (Waits until Transaction 1 completes)
|
+---------------------+
|
4 | | | Commit |
+---------------------+ +---------------------+
In this example, Transaction 1 selects orders with a 'Pending' status and prevents changes by acquiring locks on the selected data. Transaction 2, attempting to update an order with a 'Shipped' status, must wait until Transaction 1 completes and releases its locks. While Serializable ensures the highest level of consistency, it can introduce delays due to locking mechanisms, affecting system performance.
Summary:
Here's a table summarizing the differences, pros, and cons for the four isolation levels: Read Uncommitted (Level 0), Read Committed (Level 1), Repeatable Read (Level 2), and Serializable (Level 3).
Isolation Level | Description | Pros | Cons |
---|---|---|---|
Read Uncommitted (Level 0) | Transactions can read uncommitted changes made by others. | - Highest concurrency - Minimal locking |
- Dirty reads - Non-repeatable reads - Phantom reads |
Read Committed (Level 1) | Transactions can only read committed data. | - Reduced risk of dirty reads | - Non-repeatable reads - Phantom reads |
Repeatable Read (Level 2) | Once data is read, it remains the same throughout the transaction. | - Prevents dirty and non-repeatable reads | - Phantom reads |
Serializable (Level 3) | Strictest isolation, ensuring complete isolation from other transactions. | - Highest data consistency | - Reduced concurrency due to increased locking |
Read Uncommitted (Level 0):
- Pros: Highest concurrency, minimal locking.
- Cons: Dirty reads, non-repeatable reads, phantom reads.
Read Committed (Level 1):
- Pros: Reduced risk of dirty reads.
- Cons: Non-repeatable reads, phantom reads.
Repeatable Read (Level 2):
- Pros: Prevents dirty and non-repeatable reads.
- Cons: Phantom reads.
Serializable (Level 3):
- Pros: Highest data consistency.
- Cons: Reduced concurrency due to increased locking.
Choosing the Right Isolation Level
Consider transaction requirements, concurrency needs, application type, and performance considerations to strike the right balance for optimal data consistency and system performance in your database transactions. Understanding isolation levels enables informed decision-making tailored to your application's specific needs.