Top 10 Node.js Interview Questions (2024 Edition) Part 1
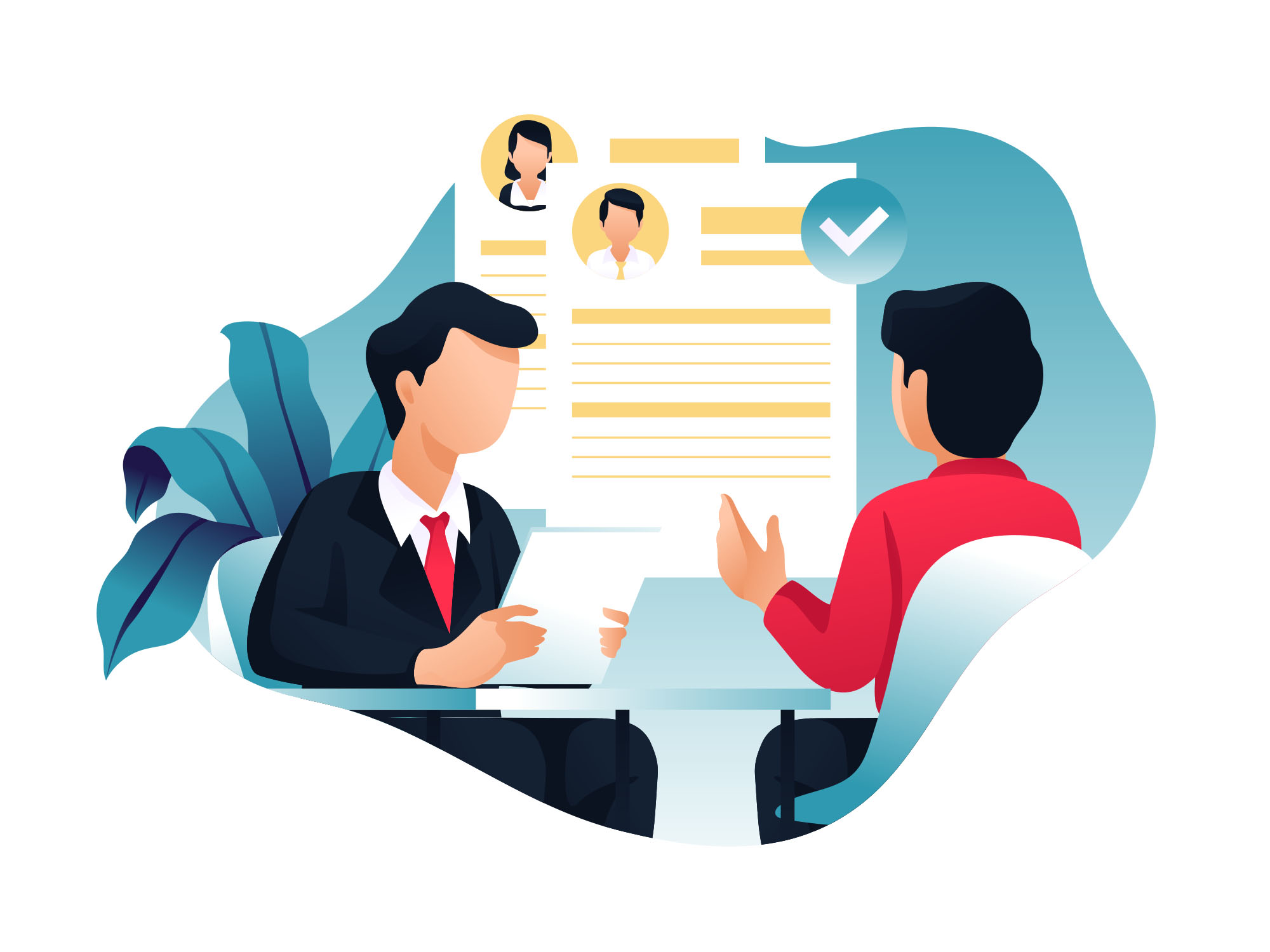
Introduction
Embark on a thrilling journey into the heart of Node.js, where innovation meets curiosity and possibilities are as boundless as your imagination. In this insightful blog, we dive into the latest and greatest of Node.js, unveiling the top 10 interview questions of 2024. Whether you're a seasoned developer, a curious tech enthusiast, or someone eager to explore the dynamic world of JavaScript, this blog is your compass through the ever-evolving landscape of Node.js. Join us as we decode the intricacies, uncover the secrets, and pave the way for a future shaped by the power of Node.js. Let the adventure unfold, and let your curiosity be the guide!
1. How does Node.js differ from other server-side technologies like Apache or Django?
Node.js, Apache, and Django are all technologies used for server-side development, but they have different architectures, use cases, and programming languages. Here's a brief comparison:
Node.js:
- Language: JavaScript
- Architecture: Event-driven, non-blocking I/O
- Use Case: Designed for building scalable network applications. Particularly good for real-time applications like chat applications and online gaming.
- Example:
// Simple HTTP server using Node.js const http = require('http'); const server = http.createServer((req, res) => { res.writeHead(200, { 'Content-Type': 'text/plain' }); res.end('Hello, Node.js!'); }); server.listen(3000, '127.0.0.1', () => { console.log('Server listening on port 3000'); });
Apache:
- Language: Typically configured using a combination of Apache configuration directives and scripting languages like PHP, Python, or others.
- Architecture: Multi-process, multi-threaded
- Use Case: General-purpose web server suitable for a wide range of applications. Often used in combination with PHP, Python, or other server-side scripting languages.
- Example:
# Apache configuration to serve a PHP file <Files "index.php"> AddType application/x-httpd-php .php Action application/x-httpd-php "/php-cgi-path/php-cgi" </Files>
Django:
- Language: Python
- Architecture: Model-View-Controller (MVC) or Model-View-Template (MVT)
- Use Case: High-level web framework for building web applications quickly and efficiently. It includes an ORM (Object-Relational Mapping) system for database interaction.
- Example:
# A simple Django view that renders a template from django.http import HttpResponse from django.shortcuts import render def hello(request): context = {'message': 'Hello, Django!'} return render(request, 'hello.html', context)
In summary, Node.js is known for its event-driven, non-blocking I/O model and is well-suited for real-time applications. Apache is a versatile web server often used with various scripting languages, while Django is a high-level Python web framework designed for rapid development of web applications. The choice between them depends on the specific requirements and preferences of the project at hand.
2. Explain the role of the V8 engine in Node.js.
The V8 engine is a critical component in the Node.js runtime, responsible for executing JavaScript code. Developed by Google, the V8 engine is an open-source, high-performance JavaScript engine used in Google Chrome and other Chromium-based browsers. Node.js leverages the V8 engine to execute JavaScript code on the server-side.
Here's an overview of the role of the V8 engine in Node.js:
JavaScript Execution:
- The V8 engine compiles JavaScript code into machine code for efficient execution on the server.
- It uses Just-In-Time (JIT) compilation to convert JavaScript source code into native machine code, optimizing performance.
Event Loop:
- Node.js is designed to be non-blocking and asynchronous, and the V8 engine plays a key role in this aspect.
- The event-driven, non-blocking I/O model of Node.js is powered by the event loop, and the V8 engine ensures efficient handling of asynchronous operations.
Example:
Consider a simple Node.js script that uses the V8 engine to execute JavaScript code. Below is a basic example of a Node.js script:
// Simple Node.js script using the V8 engine // This function demonstrates asynchronous behavior using setTimeout function asyncFunction(callback) { setTimeout(() => { console.log('Async operation completed'); callback(); }, 1000); } // Main function function main() { console.log('Start of script'); // Call the asynchronous function asyncFunction(() => { console.log('End of script'); }); } // Run the main function main();
In this example, the
asyncFunction
simulates an asynchronous operation usingsetTimeout
. The V8 engine handles the asynchronous nature of the code, allowing other operations to continue while waiting for the timeout to complete. The event loop, powered by V8, ensures that the script remains responsive and non-blocking.
The V8 engine's efficiency in handling JavaScript execution and its support for asynchronous operations contribute to Node.js's ability to build scalable and high-performance server-side applications.
3. Differentiate between process.nextTick and setImmediate.
process.nextTick
and setImmediate
are both used in Node.js for scheduling the execution of callback functions, but they have some differences in terms of when the callbacks are executed within the event loop.
process.nextTick
:process.nextTick
queues the callback to be executed in the next iteration of the event loop, before any I/O events.- It's often used to defer the execution of a callback until the current operation completes, allowing the event loop to continue processing other tasks.
Example:
console.log('Start of script'); process.nextTick(() => { console.log('Inside process.nextTick callback'); }); console.log('End of script');
In this example, the
process.nextTick
callback will be executed in the next iteration of the event loop, after the current script has finished execution. The output will be:Start of script End of script Inside process.nextTick callback
setImmediate
:setImmediate
queues the callback to be executed in the next iteration of the event loop, but after I/O events. It's often used to execute callbacks after the current event loop cycle, allowing I/O events to be processed first.
Example:
console.log('Start of script'); setImmediate(() => { console.log('Inside setImmediate callback'); }); console.log('End of script');
In this example, the
setImmediate
callback will be executed in the next iteration of the event loop, after the current script has finished execution and after any pending I/O events. The output will be:Start of script End of script Inside setImmediate callback
In summary, both process.nextTick
and setImmediate
are used for scheduling callbacks in the next iteration of the event loop, but process.nextTick
executes before I/O events, while setImmediate
executes after I/O events. Choosing between them depends on the specific requirements of your code and when you want the callback to be executed in relation to other tasks in the event loop.
4. How does Node.js handle concurrency?
Node.js handles concurrency through its event-driven, non-blocking I/O model. It uses an event loop to manage asynchronous operations efficiently, allowing it to handle a large number of simultaneous connections without the need for threads or processes for each connection. Here's an overview of how Node.js handles concurrency:
Event Loop:
- Node.js employs a single-threaded event loop, which is the core of its concurrency model.
- The event loop continuously checks for events (such as I/O operations or timers) in a non-blocking manner.
- When an event occurs, the associated callback function is queued to be executed.
Non-Blocking I/O:
- Node.js uses non-blocking I/O operations, which means that it doesn't wait for a task (like reading from a file or making a network request) to complete before moving on to the next task.
- Instead, it initiates the task and continues processing other tasks, relying on callbacks to handle the results when the I/O operation completes.
Example:
// Example of non-blocking I/O in Node.js const fs = require('fs'); console.log('Start of script'); // Asynchronous file read operation fs.readFile('example.txt', 'utf8', (err, data) => { if (err) { console.error(err); return; } console.log('File content:', data); }); console.log('End of script');
In this example, the
readFile
function initiates a file read operation asynchronously. Instead of waiting for the file read to complete, the event loop continues to the next statement (console.log('End of script')
). When the file read operation is finished, the callback function is pushed to the event loop's queue and executed. This asynchronous, non-blocking approach allows Node.js to efficiently handle multiple I/O operations simultaneously.Concurrency with Event Emitters:
- Node.js also utilizes event emitters to handle concurrency. Modules like
EventEmitter
allow developers to create custom events and listeners, enabling effective communication between different parts of an application.
const EventEmitter = require('events'); // Example of using Event Emitters for concurrency class MyEmitter extends EventEmitter {} const myEmitter = new MyEmitter(); myEmitter.on('customEvent', () => { console.log('Custom event fired'); }); // Emit the custom event asynchronously setImmediate(() => { myEmitter.emit('customEvent'); });
Here, the custom event is emitted asynchronously using
setImmediate
, demonstrating how event emitters contribute to concurrency in Node.js.- Node.js also utilizes event emitters to handle concurrency. Modules like
By combining the event loop, non-blocking I/O, and event emitters, Node.js achieves efficient concurrency, making it well-suited for handling a large number of simultaneous connections in applications such as web servers or real-time applications.
5. Describe the purpose of the 'events' module in Node.js.
The events
module in Node.js provides an event-driven programming paradigm by allowing objects to emit events and register listeners for those events. This module is crucial for building applications that respond to asynchronous and event-driven scenarios. It is based on the observer pattern, where objects (emitters) maintain a list of dependent objects (listeners) and notify them of any state changes.
Here's an overview of the purpose of the events
module and an example:
Purpose of the events
module:
Event Emitter:
- The
events
module provides theEventEmitter
class, which serves as the foundation for creating objects that can emit events.
- The
Event Handling:
- Objects that inherit from
EventEmitter
can emit named events. Other objects (listeners) can register functions (event handlers) to be executed when a specific event occurs.
- Objects that inherit from
Asynchronous Communication:
- The
events
module facilitates asynchronous communication between different parts of a program, making it suitable for scenarios where actions in one part of the program should trigger reactions in another part.
- The
Example:
// Example using the 'events' module in Node.js
const EventEmitter = require('events');
// Create a custom event emitter
class MyEmitter extends EventEmitter {}
// Create an instance of the custom emitter
const myEmitter = new MyEmitter();
// Register an event handler for the 'customEvent' event
myEmitter.on('customEvent', (arg) => {
console.log(`Custom event occurred with argument: ${arg}`);
});
// Emit the 'customEvent' event with an argument asynchronously
setImmediate(() => {
myEmitter.emit('customEvent', 'Hello, EventEmitter!');
});
console.log('End of script');
In this example:
- We create a custom
MyEmitter
class that extendsEventEmitter
. - An instance of
MyEmitter
is created withconst myEmitter = new MyEmitter();
. - We register an event handler for the 'customEvent' event using
myEmitter.on('customEvent', ...)
. The event handler logs the provided argument. - Using
setImmediate
, we emit the 'customEvent' event with the argument 'Hello, EventEmitter!'.
When the 'customEvent' event is emitted asynchronously, the registered event handler is called, and the message is logged. The output of the script will be:
End of script
Custom event occurred with argument: Hello, EventEmitter!
This demonstrates the basic usage of the events
module, allowing objects to communicate asynchronously by emitting and handling events. The event-driven architecture provided by this module is particularly useful for building scalable and modular applications in Node.js.
6. What is the significance of the 'util' module in Node.js?
The util
module in Node.js provides a set of utility functions that are helpful for various tasks, including debugging, inheritance, and asynchronous programming. It offers a collection of commonly used functionalities that don't fit neatly into other modules but are still important for developers. Some of the key features and functions provided by the util
module include:
Debugging and Inspection:
util.inspect
: Converts any JavaScript object into a string representation, facilitating debugging and logging.
Inheritance:
util.inherits
: Used to inherit the prototype methods and properties from one constructor into another. This function is used in classical inheritance patterns.
Promisify:
util.promisify
: Converts callback-based functions into Promises. This is particularly useful when working with asynchronous code and simplifies the use of modern asynchronous patterns.
Deprecation Warnings:
util.deprecate
: Marks a function as deprecated, and provides a warning when the deprecated function is called.
Error Handling:
util.format
: Similar toprintf
in C, it provides string formatting capabilities.
EventEmitter Enhancement:
util.inspect.defaultOptions
: Provides default options for theutil.inspect
function.util.inspect.custom
: A symbol key used to define custominspect
methods on objects.
Other Utilities:
util.isArray
,util.isDate
,util.isRegExp
, etc.: Type-checking functions for common JavaScript types.
Here's a brief example demonstrating the use of util.promisify
:
const util = require('util');
const fs = require('fs');
// Using util.promisify to convert fs.readFile into a Promise-based function
const readFileAsync = util.promisify(fs.readFile);
// Example usage
readFileAsync('example.txt', 'utf8')
.then(data => {
console.log('File content:', data);
})
.catch(error => {
console.error('Error reading file:', error);
});
In this example, util.promisify
is used to convert the callback-based fs.readFile
function into a Promise-based function (readFileAsync
). This makes it easier to work with asynchronous code using modern JavaScript features like async/await.
In summary, the util
module in Node.js provides essential utilities for various tasks, making development more convenient and efficient. Developers often leverage these functions to handle common scenarios and improve the robustness of their applications.
7. How can you handle errors in asynchronous code in Node.js?
Handling errors in asynchronous code in Node.js involves using mechanisms such as callbacks, Promises, or async/await along with try-catch blocks. Here's how you can handle errors using these approaches:
1. Callbacks:
const fs = require('fs');
fs.readFile('example.txt', 'utf8', (err, data) => {
if (err) {
console.error('Error reading file:', err);
return;
}
console.log('File content:', data);
});
In callback-style code, it's a common practice to check for errors in the first parameter of the callback function.
2. Promises:
const fs = require('fs').promises;
fs.readFile('example.txt', 'utf8')
.then(data => {
console.log('File content:', data);
})
.catch(error => {
console.error('Error reading file:', error);
});
With Promises, you can use the .then()
method to handle the successful result and the .catch()
method to handle errors.
3. Async/Await:
async function readFileAsync() {
try {
const data = await fs.promises.readFile('example.txt', 'utf8');
console.log('File content:', data);
} catch (error) {
console.error('Error reading file:', error);
}
}
readFileAsync();
Async/await is a more concise and readable way to handle asynchronous code. The try
block contains the code that may throw an error, and the catch
block handles any errors that occur.
4. Event Emitters:
When working with event emitters, you can use the error event to handle errors:
const EventEmitter = require('events');
const myEmitter = new EventEmitter();
myEmitter.on('error', (err) => {
console.error('Error:', err);
});
// Emitting an error event
myEmitter.emit('error', new Error('Something went wrong'));
5. Global Error Handling:
You can also set up a global error handler for unhandled Promise rejections or uncaught exceptions:
process.on('unhandledRejection', (reason, promise) => {
console.error('Unhandled Rejection at:', promise, 'reason:', reason);
// Handle the error or log it as needed
});
process.on('uncaughtException', (error) => {
console.error('Uncaught Exception:', error);
// Handle the error or log it as needed
});
// Example of unhandled rejection
Promise.reject(new Error('Unhandled Rejection Example'));
Please note that using global error handlers for unhandled rejections and uncaught exceptions is considered a best practice, but it's crucial to handle errors at a more granular level within your application whenever possible.
Choose the approach that best fits your code structure and coding style. Each method provides a way to gracefully handle errors and prevents them from crashing the entire Node.js process.
8. What is the purpose of the 'cluster' module, and how does it enhance Node.js performance?
The cluster
module in Node.js is designed to improve performance and scalability by enabling the creation of multiple processes, each running its own instance of the Node.js event loop. This allows a Node.js application to take full advantage of multi-core systems and distribute the processing load across multiple CPUs.
Key Purposes and Features of the cluster
module:
Forking Worker Processes:
- The
cluster
module allows you to create a master process and multiple worker processes, also known as "workers." - Each worker process runs its own instance of the Node.js event loop.
- The
Load Balancing:
- Incoming connections can be distributed among the worker processes to achieve load balancing. The master process manages the distribution of incoming requests among the workers.
Shared Server Ports:
- The master process listens on a specific port, and it forwards incoming connections to the available worker processes, allowing multiple processes to share the same server port.
Automatic Process Management:
- The
cluster
module provides automatic process management, including restarting crashed workers. If a worker dies unexpectedly, the master process can restart it.
- The
Improved Reliability and Fault Tolerance:
- If one worker encounters an error or crashes, the remaining workers can continue to handle incoming requests. This enhances the overall reliability and fault tolerance of the application.
Example Usage:
Here is a simple example demonstrating the use of the cluster
module to create a multi-process Node.js server:
const cluster = require('cluster');
const http = require('http');
const numCPUs = require('os').cpus().length;
if (cluster.isMaster) {
console.log(`Master process ${process.pid} is running`);
// Fork workers
for (let i = 0; i < numCPUs; i++) {
cluster.fork();
}
// Handle worker exit event
cluster.on('exit', (worker, code, signal) => {
console.log(`Worker ${worker.process.pid} died with code ${code} and signal ${signal}`);
// Restart the worker
cluster.fork();
});
} else {
// Worker processes run the actual server logic
const server = http.createServer((req, res) => {
res.writeHead(200);
res.end('Hello, World!\n');
});
server.listen(3000, () => {
console.log(`Worker ${process.pid} is listening on port 3000`);
});
}
In this example:
- The master process (identified by
cluster.isMaster
) forks multiple worker processes. - Each worker process runs its own HTTP server.
- If a worker process dies, the master process restarts it, ensuring the application's continued availability.
Enhancing Performance:
The cluster
module enhances Node.js performance by taking advantage of multiple CPU cores. With a separate event loop for each worker process, the application can handle more concurrent connections and distribute the processing load, leading to improved performance and responsiveness.
It's important to note that the cluster
module is most effective for applications that are I/O-bound or can benefit from parallel processing. For CPU-bound tasks, other approaches such as using worker threads or a message-passing model may be more suitable.
9. What is npm audit, and why is it important in Node.js development?
npm audit
is a command-line tool provided by npm (Node Package Manager) that helps developers identify and fix security vulnerabilities in their Node.js projects by analyzing the project's dependencies. It is an essential part of the npm ecosystem for ensuring the security of your Node.js applications.
Key features of npm audit
:
Vulnerability Scanning:
npm audit
scans the project's dependency tree and checks it against the npm Security Database, which contains information about known vulnerabilities in packages.
Severity Levels:
- It categorizes vulnerabilities into different severity levels, such as low, moderate, high, and critical. The severity level is an indicator of the potential impact of the vulnerability.
Detailed Reports:
npm audit
provides detailed reports that include information about the vulnerabilities found, the affected packages, and suggested actions to resolve the issues.
Fix Recommendations:
- The tool often suggests commands to automatically fix vulnerabilities by updating to a patched version of the affected packages.
Why npm audit
is important:
Security:
- Identifying and addressing security vulnerabilities is crucial for the overall security of your application. Using outdated or vulnerable dependencies can expose your application to potential threats and attacks.
Compliance:
- In many industries, compliance standards require regular security assessments and the prompt remediation of known vulnerabilities.
npm audit
helps developers meet these compliance requirements.
- In many industries, compliance standards require regular security assessments and the prompt remediation of known vulnerabilities.
Automatic Fixing:
npm audit
not only identifies vulnerabilities but also suggests commands to automatically fix the issues by updating to a version of the package that addresses the security concerns. This simplifies the process of addressing vulnerabilities.
Best Practices:
- Regularly running
npm audit
becomes a best practice in Node.js development. It helps developers stay proactive in managing security concerns and ensures that the project dependencies are up to date with the latest security patches.
- Regularly running
Usage:
To run an audit on your Node.js project, you can use the following command:
npm audit
Additionally, if you want npm
to attempt an automatic fix for reported vulnerabilities, you can use:
npm audit fix
Example Output:
$ npm audit
=== npm audit security report ===
# Run `npm audit fix` to fix 22 vulnerabilities
High Regular Expression Denial of Service
Package braces
Patched in >=3.0.1
Dependency of webpack
Path webpack > webpack-dev-server > sockjs > @ssockjs/istanbul@0.6.0
More info https://npmjs.com/advisories/786
...
found 22 vulnerabilities (1 low, 12 moderate, 7 high, 2 critical) in 1344 scanned packages
22 vulnerabilities require semver-major dependency updates.
7 vulnerabilities require manual review. See the full report for details.
In this example, the report indicates the number and severity of vulnerabilities found in the project's dependencies, along with suggestions for fixing them.
By regularly running npm audit
, developers can proactively address security issues, ensuring a more robust and secure application. It's recommended to incorporate npm audit
into your continuous integration (CI) processes to catch vulnerabilities early in the development lifecycle.
10. What is the 'Buffer' class, and when would you use it?
The Buffer
class in Node.js is a built-in class that provides a way to work with binary data directly in memory. It is particularly useful when dealing with I/O operations, network protocols, cryptography, and other scenarios where raw binary data manipulation is required. A Buffer
is essentially a fixed-size chunk of memory allocated outside the JavaScript heap, and it