Setting up Jenkins for Node.js Applications with Docker: A Step-by-Step Guide
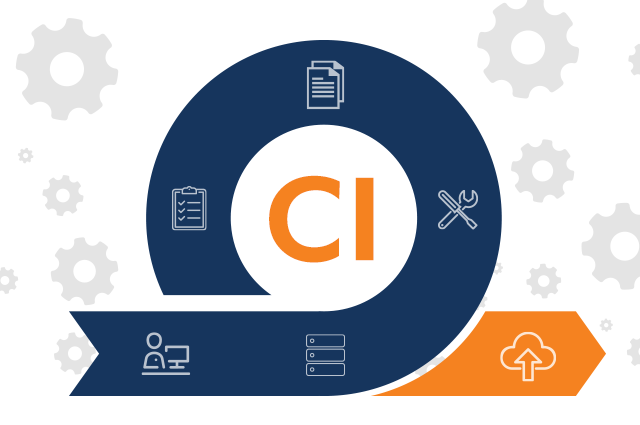
Introduction:
Continuous Integration (CI) and Continuous Deployment (CD) are crucial aspects of modern software development. Jenkins, an open-source automation server, plays a key role in automating the building, testing, and deployment of applications. In this tutorial, we'll walk through the process of setting up Jenkins for a Node.js application using Docker.
Prerequisites:
- Docker installed on your machine.
- A Node.js application with a version specified in a
package.json
file. - Basic knowledge of Docker and Jenkins.
Step 1: Install Jenkins with Docker
docker run -p 8080:8080 -p 50000:50000 -v jenkins_home:/var/jenkins_home jenkins/jenkins:lts
This command pulls the latest Jenkins LTS (Long Term Support) image, exposes ports 8080 and 50000, and mounts a volume for Jenkins data.
Step 2: Access Jenkins Web Interface
Open your browser and navigate to http://localhost:8080
. Retrieve the initial admin password from the Jenkins container:
docker exec -it <container_id> cat /var/jenkins_home/secrets/initialAdminPassword
Follow the on-screen instructions to complete the Jenkins setup.
Step 3: Install Necessary Jenkins Plugins
Once Jenkins is set up, install the required plugins. Navigate to "Manage Jenkins" -> "Manage Plugins" -> "Available" tab. Search for and install the following plugins:
- Docker
- NodeJS
Restart Jenkins after plugin installation.
Step 4: Configure NodeJS in Jenkins
Navigate to "Manage Jenkins" -> "Global Tool Configuration." Scroll down to the "NodeJS" section and click "Add NodeJS." Provide a name and select the NodeJS installation method (you can choose to install automatically or provide a path if you've already installed it on your machine).
Step 5: Create a Jenkins Pipeline for Node.js Application
Create a new item in Jenkins (Freestyle project or Pipeline). Configure your project, and in the "Build" section, add a build step.
For a Jenkinsfile, use the following example:
pipeline {
agent any
tools {
nodejs "your_nodejs_tool_name"
}
stages {
stage('Checkout') {
steps {
git 'your_repository_url'
}
}
stage('Install Dependencies') {
steps {
sh 'npm install'
}
}
stage('Build and Test') {
steps {
sh 'npm test'
}
}
stage('Docker Build') {
steps {
sh 'docker build -t your_image_name .'
}
}
stage('Docker Push') {
steps {
withCredentials([usernamePassword(credentialsId: 'your_docker_credentials_id', passwordVariable: 'DOCKER_PASSWORD', usernameVariable: 'DOCKER_USERNAME')]) {
sh "echo $DOCKER_PASSWORD | docker login -u $DOCKER_USERNAME --password-stdin"
}
sh 'docker push your_image_name'
}
}
}
}
Adjust the placeholders with your actual values.
Step 6: Setup Docker Registry Credentials
In Jenkins, go to "Manage Jenkins" -> "Manage Credentials" -> "(global)" domain -> "Add Credentials." Add your Docker Hub or preferred registry credentials.
Conclusion:
By following these steps, you've successfully set up Jenkins for a Node.js application using Docker. This automated pipeline will streamline your development workflow, ensuring efficient building, testing, and deployment of your Node.js projects. Feel free to customize the pipeline according to your specific requirements and project structure. Happy coding!